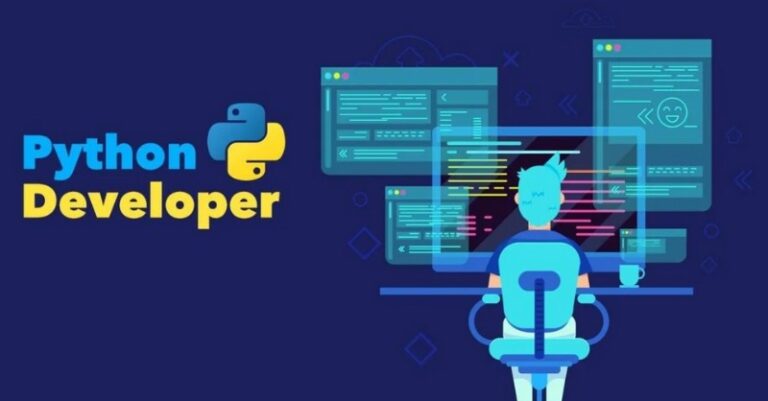
When writing code in Python, Python operators play a crucial role in performing various operations, such as arithmetic, comparisons, and logical expressions. As a developer, mastering these operators helps you write more concise, efficient, and readable code.
Understanding the types of Python operators also gives you an edge when solving coding problems or appearing for technical interviews. In this blog, we’ll explore the most important Python operators every developer should know, along with real-world examples and best practices.
Read More; How OOPs Concepts in C# Improve Software Design and Maintainability
2. What are Operators in Python?
Operators are special symbols or keywords that perform operations on variables and values. They allow developers to manipulate data, perform calculations, and make decisions within the code.
Types of Python Operators:
- Arithmetic Operators
- Comparison Operators
- Assignment Operators
- Logical Operators
- Bitwise Operators
- Membership Operators
- Identity Operators
- Ternary Operator (Conditional Expression)
- Walrus Operator
- Operator Overloading
These operators are essential to build logic for everything from simple calculations to complex programs.
3. Top 10 Python Operators Every Developer Should Know
1. Arithmetic Operators
These operators are used for basic mathematical operations.
- Operators:
+
,-
,*
,/
,//
,%
,**
- Example:pythonCopy code
result = 10 + 5 # Output: 15 power = 2 ** 3 # Output: 8 (2 raised to the power 3) print(result, power)
- Why Important:
They are fundamental in calculations and essential in any programming task.
2. Comparison Operators
These operators compare two values and return a boolean result.
- Operators:
==
,!=
,>
,<
,>=
,<=
- Example:pythonCopy code
if 10 > 5: print("10 is greater than 5") # Output: 10 is greater than 5
- Why Important:
Python operators like these are commonly used in conditional statements and loops.
3. Assignment Operators
Assignment operators assign or modify the value of a variable.
- Operators:
=
,+=
,-=
,*=
,/=
,//=
,**=
,%=
- Example:pythonCopy code
x = 10 x += 5 # Equivalent to x = x + 5 print(x) # Output: 15
- Why Important:
These operators simplify variable updates in code, making it more readable.
4. Logical Operators
Logical operators combine multiple conditions and return boolean values.
- Operators:
and
,or
,not
- Example:pythonCopy code
if True and False: print("This won't print") else: print("This will print") # Output: This will print
- Why Important:
Useful in control flow to combine multiple conditions.
5. Bitwise Operators
These operators perform operations at the binary level.
- Operators:
&
,|
,^
,~
,<<
,>>
- Example:pythonCopy code
result = 5 & 3 # Output: 1 (Bitwise AND) print(result)
- Why Important:
Bitwise operators are often used in low-level programming and optimization.
6. Membership Operators
Membership operators check if a value is part of a sequence (like lists or strings).
- Operators:
in
,not in
- Example:pythonCopy code
my_list = [1, 2, 3] print(2 in my_list) # Output: True
- Why Important:
These operators are especially useful when working with collections in Python.
7. Identity Operators
Identity operators compare the memory location of two objects.
- Operators:
is
,is not
- Example:pythonCopy code
x = [1, 2, 3] y = x print(x is y) # Output: True
- Why Important:
Type of Python operators like these are useful when comparing objects and their identities.
8. Ternary Operator (Conditional Expressions)
The ternary operator offers a shorthand way of writing conditionals.
- Syntax:
x if condition else y
- Example:pythonCopy code
result = "Even" if 4 % 2 == 0 else "Odd" print(result) # Output: Even
- Why Important:
It improves code readability by reducing the number of lines.
9. The Walrus Operator (:=
)
Introduced in Python 3.8, the Walrus operator allows assignment inside expressions.
- Example:pythonCopy code
if (length := len([1, 2, 3])) > 2: print(f"List length is {length}") # Output: List length is 3
- Why Important:
This operator makes the code more concise and readable.
10. Operator Overloading
Python allows developers to modify the behavior of operators for custom objects.
- Example:pythonCopy code
class Point: def __init__(self, x, y): self.x = x self.y = y def __add__(self, other): return Point(self.x + other.x, self.y + other.y) p1 = Point(1, 2) p2 = Point(3, 4) result = p1 + p2 # Output: 4 6 print(result.x, result.y)
- Why Important:
Operator overloading improves code flexibility and makes custom classes more intuitive.
4. Best Practices for Using Python Operators
- Don’t Overuse Bitwise Operators:
They can make code hard to read unless you document them well. - Use Membership and Identity Operators Appropriately:
Ensure you’re usingis
only for object identity comparisons and not for equality checks. - Keep Operator Overloading Simple:
Overloading should enhance, not complicate, your code.
5. Common Python Interview Questions on Operators
- What is the difference between
is
and==
? - How do
and
andor
operators work? - What is the purpose of the Walrus operator?
- How do you overload an operator in Python?
- When would you use
in
andnot in
operators?
These C# interview questions frequently appear in coding interviews, so it’s essential to understand how to use these operators effectively.
6. Conclusion
Mastering these Python operators is crucial for any developer, as they form the foundation of writing efficient, readable, and concise code. From arithmetic operations to operator overloading, each type of Python operator serves a unique purpose, helping developers build everything from basic scripts to complex applications.